RHEED Metrics with Details#
%load_ext autoreload
%autoreload 2
import os
import re
import numpy as np
import matplotlib.pyplot as plt
from m3util.viz.style import set_style
from m3util.viz.printing import printer
from m3util.viz.layout import layout_fig
from m3util.viz.text import labelfigs
from sto_rheed.Dataset import RHEED_spot_Dataset, RHEED_parameter_dataset
from sto_rheed.Viz import Viz
from sto_rheed.Fit import RHEED_image_processer, Gaussian, NormalizeData
printing_image = printer(basepath = '../Figures/1.Fit_RHEED_spot/')
printing_plot = printer(basepath = '../Figures/1.Fit_RHEED_spot/', fileformats=['png', 'svg', 'tif'], dpi=300)
set_style("printing")
printing set for seaborn
1. Download datasets from Zenodo#
# # Download the data file from Zenodo
# download=False
# if download:
# # full size version
# urls = ['https://zenodo.org/record/7950043/files/STO_STO_test6_06292022-standard.h5?download=1',
# 'https://zenodo.org/record/7950043/files/STO_STO_test7_06292022-standard.h5?download=1',
# 'https://zenodo.org/record/7950043/files/STO_STO_test9_07142022-standard.h5?download=1',
# 'https://zenodo.org/record/7950043/files/test6_gaussian_fit_parameters_all-04232023.h5?download=1',
# 'https://zenodo.org/record/7950043/files/test7_gaussian_fit_parameters_all-04232023.h5?download=1',
# 'https://zenodo.org/record/7950043/files/test9_gaussian_fit_parameters_all-04232023.h5?download=1']
# # compressed version
# urls_compressed = ['https://zenodo.org/record/7948591/files/STO_STO_test6_06292022-standard-compressed.h5?download=1',
# 'https://zenodo.org/record/7948591/files/STO_STO_test7_06292022-standard-compressed.h5?download=1',
# 'https://zenodo.org/record/7948591/files/STO_STO_test9_07142022-standard-compressed.h5?download=1',
# 'https://zenodo.org/record/7948591/files/test6_gaussian_fit_parameters_all-04232023-compressed.h5?download=1',
# 'https://zenodo.org/record/7948591/files/test7_gaussian_fit_parameters_all-04232023-compressed.h5?download=1',
# 'https://zenodo.org/record/7948591/files/test9_gaussian_fit_parameters_all-04232023-compressed.h5?download=1']
# for url in urls:
# # Specify the filename and the path to save the file
# filename = re.split(r'\?', os.path.basename(url))[0]
# save_path = './../2023_RHEED_PLD_SrTiO3/Datasets/'
# # download the file
# download_and_unzip(filename, url, save_path)
2. Fitting the RHEED Dataset#
2.1 Sample treated_213nm#
2.1.1 Loading the Dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/STO_STO_test6_06292022-standard.h5'
D1_spot = RHEED_spot_Dataset(path, sample_name='treated_213nm')
D1_spot.data_info
Growth: background_with_heater, Size of data: f(2251, 300, 300)
Growth: background_without_heater, Size of data: f(2942, 300, 300)
Growth: growth_1, Size of data: f(13189, 300, 300)
Growth: growth_10, Size of data: f(12336, 300, 300)
Growth: growth_11, Size of data: f(12799, 300, 300)
Growth: growth_12, Size of data: f(12563, 300, 300)
Growth: growth_2, Size of data: f(12858, 300, 300)
Growth: growth_3, Size of data: f(11392, 300, 300)
Growth: growth_4, Size of data: f(13067, 300, 300)
Growth: growth_5, Size of data: f(12619, 300, 300)
Growth: growth_6, Size of data: f(12610, 300, 300)
Growth: growth_7, Size of data: f(12431, 300, 300)
Growth: growth_8, Size of data: f(12553, 300, 300)
Growth: growth_9, Size of data: f(12568, 300, 300)
2.1.2 Locate the diffraction spots#
D1_spot.viz_RHEED_spot(growth='growth_2', index=0, figsize=(2,4), filename=True, printing=printing_image)
Fig. a: RHEED spot image for growth_2 at index 0.
../Figures/1.Fit_RHEED_spot/RHEED_treated_213nm_growth_2_0.png
../Figures/1.Fit_RHEED_spot/RHEED_treated_213nm_growth_2_0.svg

2.1.3 Visualize an example#
crop_dict = {'spot_1': {'y_start':40, 'y_end':80, 'x_start':100, 'x_end':160},
'spot_2': {'y_start':137, 'y_end':166, 'x_start':147, 'x_end':195},
'spot_3': {'y_start':230, 'y_end':262, 'x_start':115, 'x_end':180}}
fit_function = Gaussian().recreate_gaussian
processor = RHEED_image_processer(D1_spot, crop_dict, fit_function)
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_1', frame=0)
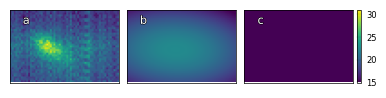
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 0.
The Gaussian fitted parameters are: img_sum=48241.00, img_max=31.00, img_mean=20.10,
img_rec_sum=48030.96, img_rec_max=22.65, img_rec_mean=20.01,
height=22.65, x=22.31, y=26.81, width_x=30.30, width_y_max=57.73.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_1', frame=0)
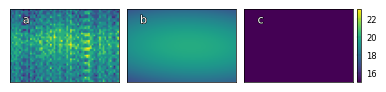
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 0.
The Gaussian fitted parameters are: img_sum=45531.00, img_max=23.00, img_mean=18.97,
img_rec_sum=45440.48, img_rec_max=19.94, img_rec_mean=18.93,
height=19.94, x=20.29, y=34.92, width_x=42.13, width_y_max=114.21.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_2', frame=0)
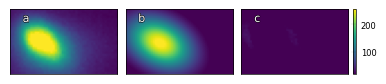
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 0.
The Gaussian fitted parameters are: img_sum=120241.00, img_max=255.00, img_mean=86.38,
img_rec_sum=105894.35, img_rec_max=260.62, img_rec_mean=76.07,
height=260.95, x=17.81, y=19.75, width_x=7.32, width_y_max=10.65.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_2', frame=0)
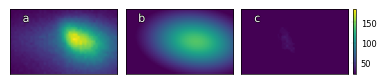
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 0.
The Gaussian fitted parameters are: img_sum=87657.00, img_max=183.00, img_mean=62.97,
img_rec_sum=82424.84, img_rec_max=139.73, img_rec_mean=59.21,
height=139.78, x=14.58, y=31.86, width_x=8.56, width_y_max=14.55.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_3', frame=0)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 0.
The Gaussian fitted parameters are: img_sum=47740.00, img_max=36.00, img_mean=22.95,
img_rec_sum=47200.00, img_rec_max=27.82, img_rec_mean=22.69,
height=27.83, x=14.75, y=19.52, width_x=21.56, width_y_max=47.67.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_3', frame=0)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 0.
The Gaussian fitted parameters are: img_sum=49480.00, img_max=34.00, img_mean=23.79,
img_rec_sum=49086.48, img_rec_max=27.89, img_rec_mean=23.60,
height=27.90, x=13.87, y=44.06, width_x=20.56, width_y_max=62.86.
2.1.4 Exam the dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/test6_gaussian_fit_parameters_all-04232023.h5'
D1_para = RHEED_parameter_dataset(path, camera_freq=500, sample_name='treated_213nm')
# D1_para.data_info
D1_para.viz_RHEED_parameter(growth='growth_1', spot='spot_2', index=0, filename = True, printing=printing_image)
../Figures/1.Fit_RHEED_spot/RHEED_treated_213nm_growth_1_spot_2_0_img,img_rec,differerce.png
../Figures/1.Fit_RHEED_spot/RHEED_treated_213nm_growth_1_spot_2_0_img,img_rec,differerce.svg
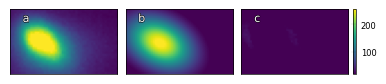
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 0.
img_sum=120241.00, img_max=255.00, img_mean=86.38
img_rec_sum=105894.35, img_rec_max=260.62, img_rec_mean=76.07
height=260.95, x=17.81, y=19.75, width_x=7.32, width_y_max=10.65
D1_para.viz_RHEED_parameter(growth='growth_1', spot='spot_2', index=5000)
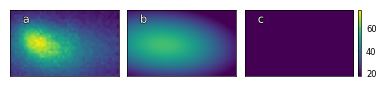
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 5000.
img_sum=53002.00, img_max=77.00, img_mean=38.08
img_rec_sum=51472.73, img_rec_max=59.34, img_rec_mean=36.98
height=59.35, x=15.42, y=16.09, width_x=11.24, width_y_max=23.73
D1_para.viz_RHEED_parameter(growth='growth_1', spot='spot_2', index=10000)
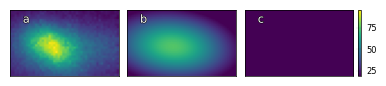
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 10000.
img_sum=60688.00, img_max=96.00, img_mean=43.60
img_rec_sum=58616.58, img_rec_max=75.27, img_rec_mean=42.11
height=75.37, x=16.06, y=20.26, width_x=10.33, width_y_max=18.64
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6',
'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
D1_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', metric_list=['img_sum', 'img_rec_sum', 'x', 'y', 'width_x', 'width_y'], head_tail=(100,500), interval=200)
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
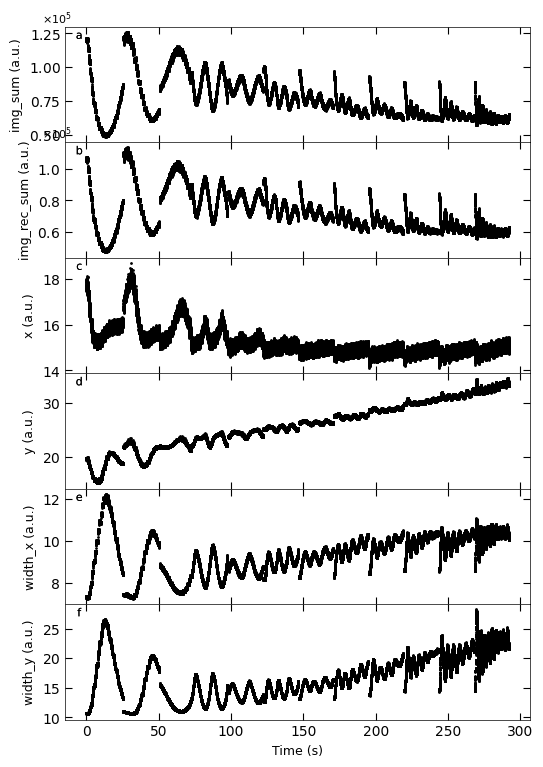
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6',
'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
D1_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', head_tail=(100,300), filename='S3-treated_213nm-RHEED_metrics', printing=printing_plot, dpi=300)
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
../Figures/1.Fit_RHEED_spot/S3-treated_213nm-RHEED_metrics.png
../Figures/1.Fit_RHEED_spot/S3-treated_213nm-RHEED_metrics.svg
../Figures/1.Fit_RHEED_spot/S3-treated_213nm-RHEED_metrics.tif
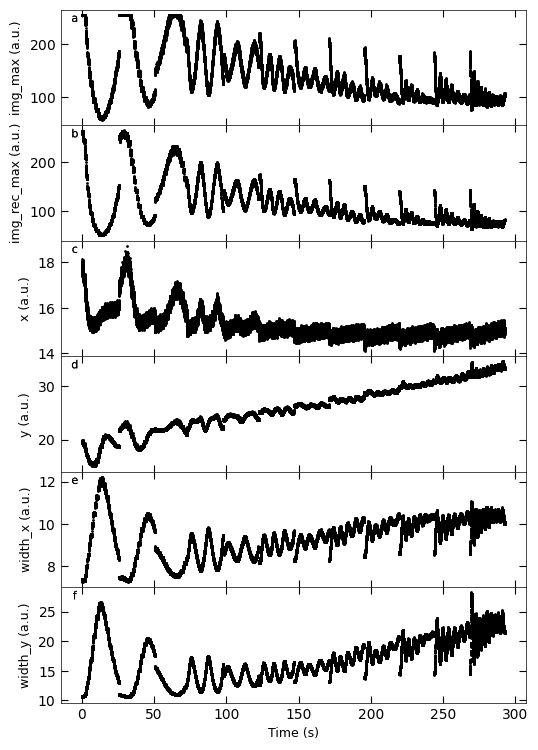
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
2.2 Sample treated_81nm#
2.2.1 Loading the Dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/STO_STO_test7_06292022-standard.h5'
D2_spot = RHEED_spot_Dataset(path, sample_name='treated_81nm')
D2_spot.data_info
Growth: background_with_heater, Size of data: f(2651, 300, 600)
Growth: background_without_heater, Size of data: f(2652, 300, 600)
Growth: growth_1, Size of data: f(8603, 300, 600)
Growth: growth_10, Size of data: f(9439, 300, 600)
Growth: growth_11, Size of data: f(12155, 300, 600)
Growth: growth_12, Size of data: f(10736, 300, 600)
Growth: growth_2, Size of data: f(10612, 300, 600)
Growth: growth_3, Size of data: f(11648, 300, 600)
Growth: growth_4, Size of data: f(12152, 300, 600)
Growth: growth_5, Size of data: f(12317, 300, 600)
Growth: growth_6, Size of data: f(12453, 300, 600)
Growth: growth_7, Size of data: f(9482, 300, 600)
Growth: growth_8, Size of data: f(9490, 300, 600)
Growth: growth_9, Size of data: f(9704, 300, 600)
2.2.2 Locate the diffraction spots#
D2_spot.viz_RHEED_spot(growth='growth_2', index=10, figsize=(2,3), filename=True, printing=printing_image)
Fig. a: RHEED spot image for growth_2 at index 10.
../Figures/1.Fit_RHEED_spot/RHEED_treated_81nm_growth_2_10.png
../Figures/1.Fit_RHEED_spot/RHEED_treated_81nm_growth_2_10.svg

2.2.3 Visualize an example#
crop_dict = {'spot_1': {'y_start':50, 'y_end':80, 'x_start':340, 'x_end':450},
'spot_2': {'y_start':143, 'y_end':165, 'x_start':415, 'x_end':455},
'spot_3': {'y_start':220, 'y_end':260, 'x_start':340, 'x_end':450}}
fit_function = Gaussian().recreate_gaussian
processor = RHEED_image_processer(D2_spot, crop_dict, fit_function)
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_1', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=65540.00, img_max=26.00, img_mean=19.86,
img_rec_sum=65251.70, img_rec_max=21.53, img_rec_mean=19.77,
height=21.53, x=14.19, y=51.74, width_x=27.23, width_y_max=127.01.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_1', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 1000.
The Gaussian fitted parameters are: img_sum=62861.00, img_max=26.00, img_mean=19.05,
img_rec_sum=62570.65, img_rec_max=20.62, img_rec_mean=18.96,
height=20.62, x=13.23, y=45.64, width_x=29.37, width_y_max=122.00.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_2', frame=1000)
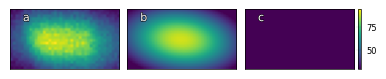
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=52423.00, img_max=94.00, img_mean=59.57,
img_rec_sum=51037.70, img_rec_max=89.54, img_rec_mean=58.00,
height=89.60, x=11.21, y=19.78, width_x=9.67, width_y_max=17.10.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_2', frame=1000)
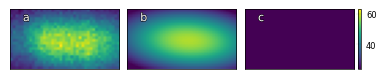
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 1000.
The Gaussian fitted parameters are: img_sum=38092.00, img_max=63.00, img_mean=43.29,
img_rec_sum=37432.69, img_rec_max=58.39, img_rec_mean=42.54,
height=58.39, x=11.58, y=22.47, width_x=10.38, width_y_max=23.31.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_3', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=87305.00, img_max=27.00, img_mean=19.84,
img_rec_sum=87167.35, img_rec_max=21.47, img_rec_mean=19.81,
height=21.47, x=24.11, y=49.98, width_x=39.04, width_y_max=130.77.
img, img_rec, parameters = processor.visualize(growth='growth_12', spot='spot_3', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_12 at index 1000.
The Gaussian fitted parameters are: img_sum=88520.00, img_max=28.00, img_mean=20.12,
img_rec_sum=88317.24, img_rec_max=22.32, img_rec_mean=20.07,
height=22.32, x=23.81, y=49.66, width_x=33.31, width_y_max=115.16.
2.2.4 Exam the dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/test7_gaussian_fit_parameters_all-04232023.h5'
D2_para = RHEED_parameter_dataset(path, camera_freq=500, sample_name='treated_81nm')
# D2_para.data_info
D2_para.viz_RHEED_parameter(growth='growth_2', spot='spot_2', index=10000, filename=True, printing=printing_image)
../Figures/1.Fit_RHEED_spot/RHEED_treated_81nm_growth_2_spot_2_10000_img,img_rec,differerce.png
../Figures/1.Fit_RHEED_spot/RHEED_treated_81nm_growth_2_spot_2_10000_img,img_rec,differerce.svg
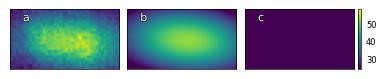
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_2 at index 10000.
img_sum=35031.00, img_max=58.00, img_mean=39.81
img_rec_sum=34451.97, img_rec_max=52.63, img_rec_mean=39.15
height=52.64, x=11.50, y=22.16, width_x=11.22, width_y_max=22.80
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
D2_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', metric_list=['img_sum', 'img_rec_sum', 'x', 'y', 'width_x', 'width_y'], )
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
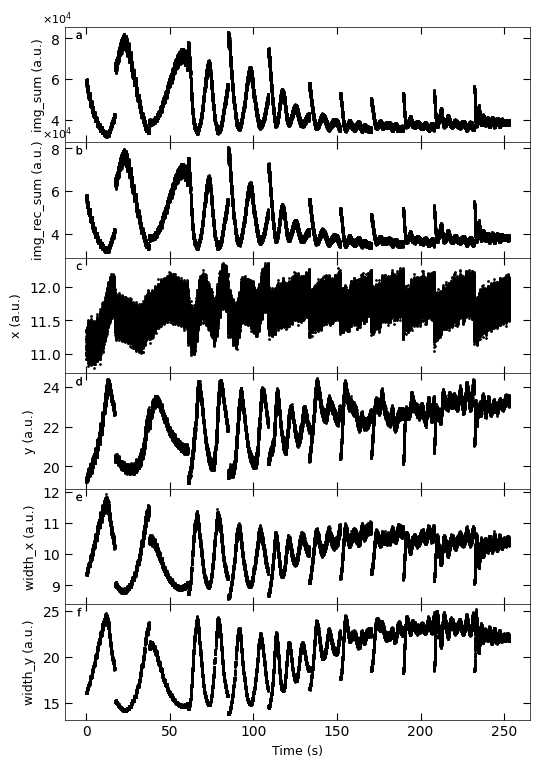
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
D2_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', filename='S4-treated_81nm-RHEED_metrics', printing=printing_plot, dpi=300)
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
../Figures/1.Fit_RHEED_spot/S4-treated_81nm-RHEED_metrics.png
../Figures/1.Fit_RHEED_spot/S4-treated_81nm-RHEED_metrics.svg
../Figures/1.Fit_RHEED_spot/S4-treated_81nm-RHEED_metrics.tif
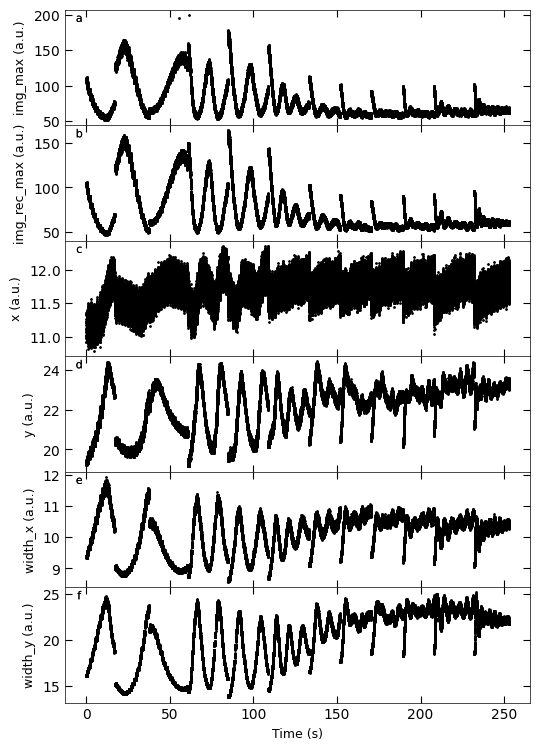
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
2.3 Sample untreated_162nm#
2.3.1 Loading the Dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/STO_STO_test9_07142022-standard.h5'
D3_spot = RHEED_spot_Dataset(path, sample_name='untreated_162nm')
D3_spot.data_info
Growth: background_with_heater, Size of data: f(2023, 300, 300)
Growth: background_without_heater, Size of data: f(3060, 300, 300)
Growth: growth_1, Size of data: f(13510, 300, 300)
Growth: growth_10, Size of data: f(12488, 300, 300)
Growth: growth_11, Size of data: f(12221, 300, 300)
Growth: growth_12, Size of data: f(12526, 300, 300)
Growth: growth_13, Size of data: f(12318, 300, 300)
Growth: growth_14, Size of data: f(12206, 300, 300)
Growth: growth_15, Size of data: f(12269, 300, 300)
Growth: growth_16, Size of data: f(12133, 300, 300)
Growth: growth_17, Size of data: f(12085, 300, 300)
Growth: growth_18, Size of data: f(12113, 300, 300)
Growth: growth_2, Size of data: f(9321, 300, 300)
Growth: growth_3, Size of data: f(12008, 300, 300)
Growth: growth_4, Size of data: f(13125, 300, 300)
Growth: growth_5, Size of data: f(12982, 300, 300)
Growth: growth_6, Size of data: f(12776, 300, 300)
Growth: growth_7, Size of data: f(12670, 300, 300)
Growth: growth_8, Size of data: f(12441, 300, 300)
Growth: growth_9, Size of data: f(12222, 300, 300)
2.3.2 Locate the diffraction spots#
D3_spot.viz_RHEED_spot(growth='growth_2', index=0, figsize=(2,4), filename=True, printing=printing_image)
Fig. a: RHEED spot image for growth_2 at index 0.
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_2_0.png
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_2_0.svg

D3_spot.viz_RHEED_spot(growth='growth_1', index=0, figsize=(2,4), label_index=0, clim=(0, 168), filename=True, printing=printing_image)
D3_spot.viz_RHEED_spot(growth='growth_1', index=13000, figsize=(2,4), label_index=1, clim=(0, 168), filename=True, printing=printing_image)
Fig. a: RHEED spot image for growth_1 at index 0.
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_1_0.png
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_1_0.svg

Fig. b: RHEED spot image for growth_1 at index 13000.
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_1_13000.png
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_1_13000.svg

2.3.3 Visualize an example#
# sets where to crop the image to extact the peaks
crop_dict = {'spot_1': {'y_start':50, 'y_end':72, 'x_start':100, 'x_end':150},
'spot_2': {'y_start':143, 'y_end':162, 'x_start':150, 'x_end':190},
'spot_3': {'y_start':218, 'y_end':262, 'x_start':100, 'x_end':165}}
fit_function = Gaussian().recreate_gaussian
processor = RHEED_image_processer(D3_spot, crop_dict, fit_function)
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_1', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=23046.00, img_max=33.00, img_mean=20.95,
img_rec_sum=22881.26, img_rec_max=24.39, img_rec_mean=20.80,
height=24.39, x=12.31, y=26.93, width_x=16.87, width_y_max=36.30.
img, img_rec, parameters = processor.visualize(growth='growth_18', spot='spot_1', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_18 at index 1000.
The Gaussian fitted parameters are: img_sum=22782.00, img_max=30.00, img_mean=20.71,
img_rec_sum=22540.11, img_rec_max=23.95, img_rec_mean=20.49,
height=23.95, x=10.74, y=23.69, width_x=15.40, width_y_max=40.17.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_2', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=58629.00, img_max=172.00, img_mean=77.14,
img_rec_sum=56051.32, img_rec_max=152.96, img_rec_mean=73.75,
height=153.25, x=11.26, y=18.91, width_x=7.66, width_y_max=11.11.
img, img_rec, parameters = processor.visualize(growth='growth_18', spot='spot_2', frame=1000)

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_18 at index 1000.
The Gaussian fitted parameters are: img_sum=35114.00, img_max=92.00, img_mean=46.20,
img_rec_sum=33973.47, img_rec_max=75.65, img_rec_mean=44.70,
height=75.75, x=10.23, y=20.99, width_x=7.94, width_y_max=14.74.
img, img_rec, parameters = processor.visualize(growth='growth_1', spot='spot_3', frame=1000)
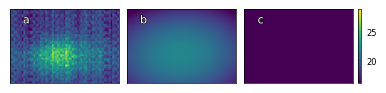
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_1 at index 1000.
The Gaussian fitted parameters are: img_sum=56802.00, img_max=29.00, img_mean=19.86,
img_rec_sum=56669.50, img_rec_max=21.97, img_rec_mean=19.81,
height=21.97, x=26.85, y=31.92, width_x=40.82, width_y_max=61.30.
img, img_rec, parameters = processor.visualize(growth='growth_18', spot='spot_3', frame=1000)
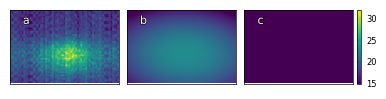
Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_18 at index 1000.
The Gaussian fitted parameters are: img_sum=57391.00, img_max=32.00, img_mean=20.07,
img_rec_sum=57205.76, img_rec_max=23.31, img_rec_mean=20.00,
height=23.31, x=26.83, y=34.85, width_x=31.40, width_y_max=54.38.
2.3.4 Examine the dataset#
path = 'D:/STO_STO-Data/RHEED/STO_STO_Berkeley/test9_gaussian_fit_parameters_all-04232023.h5'
D3_para = RHEED_parameter_dataset(path, camera_freq=500, sample_name='untreated_162nm')
D3_para.data_info
Growth: background_with_heater:
--spot: spot_1:
----height:, Size of data: (2023,)
----img_max:, Size of data: (2023,)
----img_mean:, Size of data: (2023,)
----img_rec_max:, Size of data: (2023,)
----img_rec_mean:, Size of data: (2023,)
----img_rec_sum:, Size of data: (2023,)
----img_sum:, Size of data: (2023,)
----raw_image:, Size of data: (2023, 22, 50)
----reconstructed_image:, Size of data: (2023, 22, 50)
----width_x:, Size of data: (2023,)
----width_y:, Size of data: (2023,)
----x:, Size of data: (2023,)
----y:, Size of data: (2023,)
--spot: spot_2:
----height:, Size of data: (2023,)
----img_max:, Size of data: (2023,)
----img_mean:, Size of data: (2023,)
----img_rec_max:, Size of data: (2023,)
----img_rec_mean:, Size of data: (2023,)
----img_rec_sum:, Size of data: (2023,)
----img_sum:, Size of data: (2023,)
----raw_image:, Size of data: (2023, 19, 40)
----reconstructed_image:, Size of data: (2023, 19, 40)
----width_x:, Size of data: (2023,)
----width_y:, Size of data: (2023,)
----x:, Size of data: (2023,)
----y:, Size of data: (2023,)
--spot: spot_3:
----height:, Size of data: (2023,)
----img_max:, Size of data: (2023,)
----img_mean:, Size of data: (2023,)
----img_rec_max:, Size of data: (2023,)
----img_rec_mean:, Size of data: (2023,)
----img_rec_sum:, Size of data: (2023,)
----img_sum:, Size of data: (2023,)
----raw_image:, Size of data: (2023, 44, 65)
----reconstructed_image:, Size of data: (2023, 44, 65)
----width_x:, Size of data: (2023,)
----width_y:, Size of data: (2023,)
----x:, Size of data: (2023,)
----y:, Size of data: (2023,)
Growth: background_without_heater:
--spot: spot_1:
----height:, Size of data: (3060,)
----img_max:, Size of data: (3060,)
----img_mean:, Size of data: (3060,)
----img_rec_max:, Size of data: (3060,)
----img_rec_mean:, Size of data: (3060,)
----img_rec_sum:, Size of data: (3060,)
----img_sum:, Size of data: (3060,)
----raw_image:, Size of data: (3060, 22, 50)
----reconstructed_image:, Size of data: (3060, 22, 50)
----width_x:, Size of data: (3060,)
----width_y:, Size of data: (3060,)
----x:, Size of data: (3060,)
----y:, Size of data: (3060,)
--spot: spot_2:
----height:, Size of data: (3060,)
----img_max:, Size of data: (3060,)
----img_mean:, Size of data: (3060,)
----img_rec_max:, Size of data: (3060,)
----img_rec_mean:, Size of data: (3060,)
----img_rec_sum:, Size of data: (3060,)
----img_sum:, Size of data: (3060,)
----raw_image:, Size of data: (3060, 19, 40)
----reconstructed_image:, Size of data: (3060, 19, 40)
----width_x:, Size of data: (3060,)
----width_y:, Size of data: (3060,)
----x:, Size of data: (3060,)
----y:, Size of data: (3060,)
--spot: spot_3:
----height:, Size of data: (3060,)
----img_max:, Size of data: (3060,)
----img_mean:, Size of data: (3060,)
----img_rec_max:, Size of data: (3060,)
----img_rec_mean:, Size of data: (3060,)
----img_rec_sum:, Size of data: (3060,)
----img_sum:, Size of data: (3060,)
----raw_image:, Size of data: (3060, 44, 65)
----reconstructed_image:, Size of data: (3060, 44, 65)
----width_x:, Size of data: (3060,)
----width_y:, Size of data: (3060,)
----x:, Size of data: (3060,)
----y:, Size of data: (3060,)
Growth: growth_1:
--spot: spot_1:
----height:, Size of data: (13510,)
----img_max:, Size of data: (13510,)
----img_mean:, Size of data: (13510,)
----img_rec_max:, Size of data: (13510,)
----img_rec_mean:, Size of data: (13510,)
----img_rec_sum:, Size of data: (13510,)
----img_sum:, Size of data: (13510,)
----raw_image:, Size of data: (13510, 22, 50)
----reconstructed_image:, Size of data: (13510, 22, 50)
----width_x:, Size of data: (13510,)
----width_y:, Size of data: (13510,)
----x:, Size of data: (13510,)
----y:, Size of data: (13510,)
--spot: spot_2:
----height:, Size of data: (13510,)
----img_max:, Size of data: (13510,)
----img_mean:, Size of data: (13510,)
----img_rec_max:, Size of data: (13510,)
----img_rec_mean:, Size of data: (13510,)
----img_rec_sum:, Size of data: (13510,)
----img_sum:, Size of data: (13510,)
----raw_image:, Size of data: (13510, 19, 40)
----reconstructed_image:, Size of data: (13510, 19, 40)
----width_x:, Size of data: (13510,)
----width_y:, Size of data: (13510,)
----x:, Size of data: (13510,)
----y:, Size of data: (13510,)
--spot: spot_3:
----height:, Size of data: (13510,)
----img_max:, Size of data: (13510,)
----img_mean:, Size of data: (13510,)
----img_rec_max:, Size of data: (13510,)
----img_rec_mean:, Size of data: (13510,)
----img_rec_sum:, Size of data: (13510,)
----img_sum:, Size of data: (13510,)
----raw_image:, Size of data: (13510, 44, 65)
----reconstructed_image:, Size of data: (13510, 44, 65)
----width_x:, Size of data: (13510,)
----width_y:, Size of data: (13510,)
----x:, Size of data: (13510,)
----y:, Size of data: (13510,)
Growth: growth_10:
--spot: spot_1:
----height:, Size of data: (12488,)
----img_max:, Size of data: (12488,)
----img_mean:, Size of data: (12488,)
----img_rec_max:, Size of data: (12488,)
----img_rec_mean:, Size of data: (12488,)
----img_rec_sum:, Size of data: (12488,)
----img_sum:, Size of data: (12488,)
----raw_image:, Size of data: (12488, 22, 50)
----reconstructed_image:, Size of data: (12488, 22, 50)
----width_x:, Size of data: (12488,)
----width_y:, Size of data: (12488,)
----x:, Size of data: (12488,)
----y:, Size of data: (12488,)
--spot: spot_2:
----height:, Size of data: (12488,)
----img_max:, Size of data: (12488,)
----img_mean:, Size of data: (12488,)
----img_rec_max:, Size of data: (12488,)
----img_rec_mean:, Size of data: (12488,)
----img_rec_sum:, Size of data: (12488,)
----img_sum:, Size of data: (12488,)
----raw_image:, Size of data: (12488, 19, 40)
----reconstructed_image:, Size of data: (12488, 19, 40)
----width_x:, Size of data: (12488,)
----width_y:, Size of data: (12488,)
----x:, Size of data: (12488,)
----y:, Size of data: (12488,)
--spot: spot_3:
----height:, Size of data: (12488,)
----img_max:, Size of data: (12488,)
----img_mean:, Size of data: (12488,)
----img_rec_max:, Size of data: (12488,)
----img_rec_mean:, Size of data: (12488,)
----img_rec_sum:, Size of data: (12488,)
----img_sum:, Size of data: (12488,)
----raw_image:, Size of data: (12488, 44, 65)
----reconstructed_image:, Size of data: (12488, 44, 65)
----width_x:, Size of data: (12488,)
----width_y:, Size of data: (12488,)
----x:, Size of data: (12488,)
----y:, Size of data: (12488,)
Growth: growth_11:
--spot: spot_1:
----height:, Size of data: (12221,)
----img_max:, Size of data: (12221,)
----img_mean:, Size of data: (12221,)
----img_rec_max:, Size of data: (12221,)
----img_rec_mean:, Size of data: (12221,)
----img_rec_sum:, Size of data: (12221,)
----img_sum:, Size of data: (12221,)
----raw_image:, Size of data: (12221, 22, 50)
----reconstructed_image:, Size of data: (12221, 22, 50)
----width_x:, Size of data: (12221,)
----width_y:, Size of data: (12221,)
----x:, Size of data: (12221,)
----y:, Size of data: (12221,)
--spot: spot_2:
----height:, Size of data: (12221,)
----img_max:, Size of data: (12221,)
----img_mean:, Size of data: (12221,)
----img_rec_max:, Size of data: (12221,)
----img_rec_mean:, Size of data: (12221,)
----img_rec_sum:, Size of data: (12221,)
----img_sum:, Size of data: (12221,)
----raw_image:, Size of data: (12221, 19, 40)
----reconstructed_image:, Size of data: (12221, 19, 40)
----width_x:, Size of data: (12221,)
----width_y:, Size of data: (12221,)
----x:, Size of data: (12221,)
----y:, Size of data: (12221,)
--spot: spot_3:
----height:, Size of data: (12221,)
----img_max:, Size of data: (12221,)
----img_mean:, Size of data: (12221,)
----img_rec_max:, Size of data: (12221,)
----img_rec_mean:, Size of data: (12221,)
----img_rec_sum:, Size of data: (12221,)
----img_sum:, Size of data: (12221,)
----raw_image:, Size of data: (12221, 44, 65)
----reconstructed_image:, Size of data: (12221, 44, 65)
----width_x:, Size of data: (12221,)
----width_y:, Size of data: (12221,)
----x:, Size of data: (12221,)
----y:, Size of data: (12221,)
Growth: growth_12:
--spot: spot_1:
----height:, Size of data: (12526,)
----img_max:, Size of data: (12526,)
----img_mean:, Size of data: (12526,)
----img_rec_max:, Size of data: (12526,)
----img_rec_mean:, Size of data: (12526,)
----img_rec_sum:, Size of data: (12526,)
----img_sum:, Size of data: (12526,)
----raw_image:, Size of data: (12526, 22, 50)
----reconstructed_image:, Size of data: (12526, 22, 50)
----width_x:, Size of data: (12526,)
----width_y:, Size of data: (12526,)
----x:, Size of data: (12526,)
----y:, Size of data: (12526,)
--spot: spot_2:
----height:, Size of data: (12526,)
----img_max:, Size of data: (12526,)
----img_mean:, Size of data: (12526,)
----img_rec_max:, Size of data: (12526,)
----img_rec_mean:, Size of data: (12526,)
----img_rec_sum:, Size of data: (12526,)
----img_sum:, Size of data: (12526,)
----raw_image:, Size of data: (12526, 19, 40)
----reconstructed_image:, Size of data: (12526, 19, 40)
----width_x:, Size of data: (12526,)
----width_y:, Size of data: (12526,)
----x:, Size of data: (12526,)
----y:, Size of data: (12526,)
--spot: spot_3:
----height:, Size of data: (12526,)
----img_max:, Size of data: (12526,)
----img_mean:, Size of data: (12526,)
----img_rec_max:, Size of data: (12526,)
----img_rec_mean:, Size of data: (12526,)
----img_rec_sum:, Size of data: (12526,)
----img_sum:, Size of data: (12526,)
----raw_image:, Size of data: (12526, 44, 65)
----reconstructed_image:, Size of data: (12526, 44, 65)
----width_x:, Size of data: (12526,)
----width_y:, Size of data: (12526,)
----x:, Size of data: (12526,)
----y:, Size of data: (12526,)
Growth: growth_13:
--spot: spot_1:
----height:, Size of data: (12318,)
----img_max:, Size of data: (12318,)
----img_mean:, Size of data: (12318,)
----img_rec_max:, Size of data: (12318,)
----img_rec_mean:, Size of data: (12318,)
----img_rec_sum:, Size of data: (12318,)
----img_sum:, Size of data: (12318,)
----raw_image:, Size of data: (12318, 22, 50)
----reconstructed_image:, Size of data: (12318, 22, 50)
----width_x:, Size of data: (12318,)
----width_y:, Size of data: (12318,)
----x:, Size of data: (12318,)
----y:, Size of data: (12318,)
--spot: spot_2:
----height:, Size of data: (12318,)
----img_max:, Size of data: (12318,)
----img_mean:, Size of data: (12318,)
----img_rec_max:, Size of data: (12318,)
----img_rec_mean:, Size of data: (12318,)
----img_rec_sum:, Size of data: (12318,)
----img_sum:, Size of data: (12318,)
----raw_image:, Size of data: (12318, 19, 40)
----reconstructed_image:, Size of data: (12318, 19, 40)
----width_x:, Size of data: (12318,)
----width_y:, Size of data: (12318,)
----x:, Size of data: (12318,)
----y:, Size of data: (12318,)
--spot: spot_3:
----height:, Size of data: (12318,)
----img_max:, Size of data: (12318,)
----img_mean:, Size of data: (12318,)
----img_rec_max:, Size of data: (12318,)
----img_rec_mean:, Size of data: (12318,)
----img_rec_sum:, Size of data: (12318,)
----img_sum:, Size of data: (12318,)
----raw_image:, Size of data: (12318, 44, 65)
----reconstructed_image:, Size of data: (12318, 44, 65)
----width_x:, Size of data: (12318,)
----width_y:, Size of data: (12318,)
----x:, Size of data: (12318,)
----y:, Size of data: (12318,)
Growth: growth_14:
--spot: spot_1:
----height:, Size of data: (12206,)
----img_max:, Size of data: (12206,)
----img_mean:, Size of data: (12206,)
----img_rec_max:, Size of data: (12206,)
----img_rec_mean:, Size of data: (12206,)
----img_rec_sum:, Size of data: (12206,)
----img_sum:, Size of data: (12206,)
----raw_image:, Size of data: (12206, 22, 50)
----reconstructed_image:, Size of data: (12206, 22, 50)
----width_x:, Size of data: (12206,)
----width_y:, Size of data: (12206,)
----x:, Size of data: (12206,)
----y:, Size of data: (12206,)
--spot: spot_2:
----height:, Size of data: (12206,)
----img_max:, Size of data: (12206,)
----img_mean:, Size of data: (12206,)
----img_rec_max:, Size of data: (12206,)
----img_rec_mean:, Size of data: (12206,)
----img_rec_sum:, Size of data: (12206,)
----img_sum:, Size of data: (12206,)
----raw_image:, Size of data: (12206, 19, 40)
----reconstructed_image:, Size of data: (12206, 19, 40)
----width_x:, Size of data: (12206,)
----width_y:, Size of data: (12206,)
----x:, Size of data: (12206,)
----y:, Size of data: (12206,)
--spot: spot_3:
----height:, Size of data: (12206,)
----img_max:, Size of data: (12206,)
----img_mean:, Size of data: (12206,)
----img_rec_max:, Size of data: (12206,)
----img_rec_mean:, Size of data: (12206,)
----img_rec_sum:, Size of data: (12206,)
----img_sum:, Size of data: (12206,)
----raw_image:, Size of data: (12206, 44, 65)
----reconstructed_image:, Size of data: (12206, 44, 65)
----width_x:, Size of data: (12206,)
----width_y:, Size of data: (12206,)
----x:, Size of data: (12206,)
----y:, Size of data: (12206,)
Growth: growth_15:
--spot: spot_1:
----height:, Size of data: (12269,)
----img_max:, Size of data: (12269,)
----img_mean:, Size of data: (12269,)
----img_rec_max:, Size of data: (12269,)
----img_rec_mean:, Size of data: (12269,)
----img_rec_sum:, Size of data: (12269,)
----img_sum:, Size of data: (12269,)
----raw_image:, Size of data: (12269, 22, 50)
----reconstructed_image:, Size of data: (12269, 22, 50)
----width_x:, Size of data: (12269,)
----width_y:, Size of data: (12269,)
----x:, Size of data: (12269,)
----y:, Size of data: (12269,)
--spot: spot_2:
----height:, Size of data: (12269,)
----img_max:, Size of data: (12269,)
----img_mean:, Size of data: (12269,)
----img_rec_max:, Size of data: (12269,)
----img_rec_mean:, Size of data: (12269,)
----img_rec_sum:, Size of data: (12269,)
----img_sum:, Size of data: (12269,)
----raw_image:, Size of data: (12269, 19, 40)
----reconstructed_image:, Size of data: (12269, 19, 40)
----width_x:, Size of data: (12269,)
----width_y:, Size of data: (12269,)
----x:, Size of data: (12269,)
----y:, Size of data: (12269,)
--spot: spot_3:
----height:, Size of data: (12269,)
----img_max:, Size of data: (12269,)
----img_mean:, Size of data: (12269,)
----img_rec_max:, Size of data: (12269,)
----img_rec_mean:, Size of data: (12269,)
----img_rec_sum:, Size of data: (12269,)
----img_sum:, Size of data: (12269,)
----raw_image:, Size of data: (12269, 44, 65)
----reconstructed_image:, Size of data: (12269, 44, 65)
----width_x:, Size of data: (12269,)
----width_y:, Size of data: (12269,)
----x:, Size of data: (12269,)
----y:, Size of data: (12269,)
Growth: growth_16:
--spot: spot_1:
----height:, Size of data: (12133,)
----img_max:, Size of data: (12133,)
----img_mean:, Size of data: (12133,)
----img_rec_max:, Size of data: (12133,)
----img_rec_mean:, Size of data: (12133,)
----img_rec_sum:, Size of data: (12133,)
----img_sum:, Size of data: (12133,)
----raw_image:, Size of data: (12133, 22, 50)
----reconstructed_image:, Size of data: (12133, 22, 50)
----width_x:, Size of data: (12133,)
----width_y:, Size of data: (12133,)
----x:, Size of data: (12133,)
----y:, Size of data: (12133,)
--spot: spot_2:
----height:, Size of data: (12133,)
----img_max:, Size of data: (12133,)
----img_mean:, Size of data: (12133,)
----img_rec_max:, Size of data: (12133,)
----img_rec_mean:, Size of data: (12133,)
----img_rec_sum:, Size of data: (12133,)
----img_sum:, Size of data: (12133,)
----raw_image:, Size of data: (12133, 19, 40)
----reconstructed_image:, Size of data: (12133, 19, 40)
----width_x:, Size of data: (12133,)
----width_y:, Size of data: (12133,)
----x:, Size of data: (12133,)
----y:, Size of data: (12133,)
--spot: spot_3:
----height:, Size of data: (12133,)
----img_max:, Size of data: (12133,)
----img_mean:, Size of data: (12133,)
----img_rec_max:, Size of data: (12133,)
----img_rec_mean:, Size of data: (12133,)
----img_rec_sum:, Size of data: (12133,)
----img_sum:, Size of data: (12133,)
----raw_image:, Size of data: (12133, 44, 65)
----reconstructed_image:, Size of data: (12133, 44, 65)
----width_x:, Size of data: (12133,)
----width_y:, Size of data: (12133,)
----x:, Size of data: (12133,)
----y:, Size of data: (12133,)
Growth: growth_17:
--spot: spot_1:
----height:, Size of data: (12085,)
----img_max:, Size of data: (12085,)
----img_mean:, Size of data: (12085,)
----img_rec_max:, Size of data: (12085,)
----img_rec_mean:, Size of data: (12085,)
----img_rec_sum:, Size of data: (12085,)
----img_sum:, Size of data: (12085,)
----raw_image:, Size of data: (12085, 22, 50)
----reconstructed_image:, Size of data: (12085, 22, 50)
----width_x:, Size of data: (12085,)
----width_y:, Size of data: (12085,)
----x:, Size of data: (12085,)
----y:, Size of data: (12085,)
--spot: spot_2:
----height:, Size of data: (12085,)
----img_max:, Size of data: (12085,)
----img_mean:, Size of data: (12085,)
----img_rec_max:, Size of data: (12085,)
----img_rec_mean:, Size of data: (12085,)
----img_rec_sum:, Size of data: (12085,)
----img_sum:, Size of data: (12085,)
----raw_image:, Size of data: (12085, 19, 40)
----reconstructed_image:, Size of data: (12085, 19, 40)
----width_x:, Size of data: (12085,)
----width_y:, Size of data: (12085,)
----x:, Size of data: (12085,)
----y:, Size of data: (12085,)
--spot: spot_3:
----height:, Size of data: (12085,)
----img_max:, Size of data: (12085,)
----img_mean:, Size of data: (12085,)
----img_rec_max:, Size of data: (12085,)
----img_rec_mean:, Size of data: (12085,)
----img_rec_sum:, Size of data: (12085,)
----img_sum:, Size of data: (12085,)
----raw_image:, Size of data: (12085, 44, 65)
----reconstructed_image:, Size of data: (12085, 44, 65)
----width_x:, Size of data: (12085,)
----width_y:, Size of data: (12085,)
----x:, Size of data: (12085,)
----y:, Size of data: (12085,)
Growth: growth_18:
--spot: spot_1:
----height:, Size of data: (12113,)
----img_max:, Size of data: (12113,)
----img_mean:, Size of data: (12113,)
----img_rec_max:, Size of data: (12113,)
----img_rec_mean:, Size of data: (12113,)
----img_rec_sum:, Size of data: (12113,)
----img_sum:, Size of data: (12113,)
----raw_image:, Size of data: (12113, 22, 50)
----reconstructed_image:, Size of data: (12113, 22, 50)
----width_x:, Size of data: (12113,)
----width_y:, Size of data: (12113,)
----x:, Size of data: (12113,)
----y:, Size of data: (12113,)
--spot: spot_2:
----height:, Size of data: (12113,)
----img_max:, Size of data: (12113,)
----img_mean:, Size of data: (12113,)
----img_rec_max:, Size of data: (12113,)
----img_rec_mean:, Size of data: (12113,)
----img_rec_sum:, Size of data: (12113,)
----img_sum:, Size of data: (12113,)
----raw_image:, Size of data: (12113, 19, 40)
----reconstructed_image:, Size of data: (12113, 19, 40)
----width_x:, Size of data: (12113,)
----width_y:, Size of data: (12113,)
----x:, Size of data: (12113,)
----y:, Size of data: (12113,)
--spot: spot_3:
----height:, Size of data: (12113,)
----img_max:, Size of data: (12113,)
----img_mean:, Size of data: (12113,)
----img_rec_max:, Size of data: (12113,)
----img_rec_mean:, Size of data: (12113,)
----img_rec_sum:, Size of data: (12113,)
----img_sum:, Size of data: (12113,)
----raw_image:, Size of data: (12113, 44, 65)
----reconstructed_image:, Size of data: (12113, 44, 65)
----width_x:, Size of data: (12113,)
----width_y:, Size of data: (12113,)
----x:, Size of data: (12113,)
----y:, Size of data: (12113,)
Growth: growth_2:
--spot: spot_1:
----height:, Size of data: (9321,)
----img_max:, Size of data: (9321,)
----img_mean:, Size of data: (9321,)
----img_rec_max:, Size of data: (9321,)
----img_rec_mean:, Size of data: (9321,)
----img_rec_sum:, Size of data: (9321,)
----img_sum:, Size of data: (9321,)
----raw_image:, Size of data: (9321, 22, 50)
----reconstructed_image:, Size of data: (9321, 22, 50)
----width_x:, Size of data: (9321,)
----width_y:, Size of data: (9321,)
----x:, Size of data: (9321,)
----y:, Size of data: (9321,)
--spot: spot_2:
----height:, Size of data: (9321,)
----img_max:, Size of data: (9321,)
----img_mean:, Size of data: (9321,)
----img_rec_max:, Size of data: (9321,)
----img_rec_mean:, Size of data: (9321,)
----img_rec_sum:, Size of data: (9321,)
----img_sum:, Size of data: (9321,)
----raw_image:, Size of data: (9321, 19, 40)
----reconstructed_image:, Size of data: (9321, 19, 40)
----width_x:, Size of data: (9321,)
----width_y:, Size of data: (9321,)
----x:, Size of data: (9321,)
----y:, Size of data: (9321,)
--spot: spot_3:
----height:, Size of data: (9321,)
----img_max:, Size of data: (9321,)
----img_mean:, Size of data: (9321,)
----img_rec_max:, Size of data: (9321,)
----img_rec_mean:, Size of data: (9321,)
----img_rec_sum:, Size of data: (9321,)
----img_sum:, Size of data: (9321,)
----raw_image:, Size of data: (9321, 44, 65)
----reconstructed_image:, Size of data: (9321, 44, 65)
----width_x:, Size of data: (9321,)
----width_y:, Size of data: (9321,)
----x:, Size of data: (9321,)
----y:, Size of data: (9321,)
Growth: growth_3:
--spot: spot_1:
----height:, Size of data: (12008,)
----img_max:, Size of data: (12008,)
----img_mean:, Size of data: (12008,)
----img_rec_max:, Size of data: (12008,)
----img_rec_mean:, Size of data: (12008,)
----img_rec_sum:, Size of data: (12008,)
----img_sum:, Size of data: (12008,)
----raw_image:, Size of data: (12008, 22, 50)
----reconstructed_image:, Size of data: (12008, 22, 50)
----width_x:, Size of data: (12008,)
----width_y:, Size of data: (12008,)
----x:, Size of data: (12008,)
----y:, Size of data: (12008,)
--spot: spot_2:
----height:, Size of data: (12008,)
----img_max:, Size of data: (12008,)
----img_mean:, Size of data: (12008,)
----img_rec_max:, Size of data: (12008,)
----img_rec_mean:, Size of data: (12008,)
----img_rec_sum:, Size of data: (12008,)
----img_sum:, Size of data: (12008,)
----raw_image:, Size of data: (12008, 19, 40)
----reconstructed_image:, Size of data: (12008, 19, 40)
----width_x:, Size of data: (12008,)
----width_y:, Size of data: (12008,)
----x:, Size of data: (12008,)
----y:, Size of data: (12008,)
--spot: spot_3:
----height:, Size of data: (12008,)
----img_max:, Size of data: (12008,)
----img_mean:, Size of data: (12008,)
----img_rec_max:, Size of data: (12008,)
----img_rec_mean:, Size of data: (12008,)
----img_rec_sum:, Size of data: (12008,)
----img_sum:, Size of data: (12008,)
----raw_image:, Size of data: (12008, 44, 65)
----reconstructed_image:, Size of data: (12008, 44, 65)
----width_x:, Size of data: (12008,)
----width_y:, Size of data: (12008,)
----x:, Size of data: (12008,)
----y:, Size of data: (12008,)
Growth: growth_4:
--spot: spot_1:
----height:, Size of data: (13125,)
----img_max:, Size of data: (13125,)
----img_mean:, Size of data: (13125,)
----img_rec_max:, Size of data: (13125,)
----img_rec_mean:, Size of data: (13125,)
----img_rec_sum:, Size of data: (13125,)
----img_sum:, Size of data: (13125,)
----raw_image:, Size of data: (13125, 22, 50)
----reconstructed_image:, Size of data: (13125, 22, 50)
----width_x:, Size of data: (13125,)
----width_y:, Size of data: (13125,)
----x:, Size of data: (13125,)
----y:, Size of data: (13125,)
--spot: spot_2:
----height:, Size of data: (13125,)
----img_max:, Size of data: (13125,)
----img_mean:, Size of data: (13125,)
----img_rec_max:, Size of data: (13125,)
----img_rec_mean:, Size of data: (13125,)
----img_rec_sum:, Size of data: (13125,)
----img_sum:, Size of data: (13125,)
----raw_image:, Size of data: (13125, 19, 40)
----reconstructed_image:, Size of data: (13125, 19, 40)
----width_x:, Size of data: (13125,)
----width_y:, Size of data: (13125,)
----x:, Size of data: (13125,)
----y:, Size of data: (13125,)
--spot: spot_3:
----height:, Size of data: (13125,)
----img_max:, Size of data: (13125,)
----img_mean:, Size of data: (13125,)
----img_rec_max:, Size of data: (13125,)
----img_rec_mean:, Size of data: (13125,)
----img_rec_sum:, Size of data: (13125,)
----img_sum:, Size of data: (13125,)
----raw_image:, Size of data: (13125, 44, 65)
----reconstructed_image:, Size of data: (13125, 44, 65)
----width_x:, Size of data: (13125,)
----width_y:, Size of data: (13125,)
----x:, Size of data: (13125,)
----y:, Size of data: (13125,)
Growth: growth_5:
--spot: spot_1:
----height:, Size of data: (12982,)
----img_max:, Size of data: (12982,)
----img_mean:, Size of data: (12982,)
----img_rec_max:, Size of data: (12982,)
----img_rec_mean:, Size of data: (12982,)
----img_rec_sum:, Size of data: (12982,)
----img_sum:, Size of data: (12982,)
----raw_image:, Size of data: (12982, 22, 50)
----reconstructed_image:, Size of data: (12982, 22, 50)
----width_x:, Size of data: (12982,)
----width_y:, Size of data: (12982,)
----x:, Size of data: (12982,)
----y:, Size of data: (12982,)
--spot: spot_2:
----height:, Size of data: (12982,)
----img_max:, Size of data: (12982,)
----img_mean:, Size of data: (12982,)
----img_rec_max:, Size of data: (12982,)
----img_rec_mean:, Size of data: (12982,)
----img_rec_sum:, Size of data: (12982,)
----img_sum:, Size of data: (12982,)
----raw_image:, Size of data: (12982, 19, 40)
----reconstructed_image:, Size of data: (12982, 19, 40)
----width_x:, Size of data: (12982,)
----width_y:, Size of data: (12982,)
----x:, Size of data: (12982,)
----y:, Size of data: (12982,)
--spot: spot_3:
----height:, Size of data: (12982,)
----img_max:, Size of data: (12982,)
----img_mean:, Size of data: (12982,)
----img_rec_max:, Size of data: (12982,)
----img_rec_mean:, Size of data: (12982,)
----img_rec_sum:, Size of data: (12982,)
----img_sum:, Size of data: (12982,)
----raw_image:, Size of data: (12982, 44, 65)
----reconstructed_image:, Size of data: (12982, 44, 65)
----width_x:, Size of data: (12982,)
----width_y:, Size of data: (12982,)
----x:, Size of data: (12982,)
----y:, Size of data: (12982,)
Growth: growth_6:
--spot: spot_1:
----height:, Size of data: (12776,)
----img_max:, Size of data: (12776,)
----img_mean:, Size of data: (12776,)
----img_rec_max:, Size of data: (12776,)
----img_rec_mean:, Size of data: (12776,)
----img_rec_sum:, Size of data: (12776,)
----img_sum:, Size of data: (12776,)
----raw_image:, Size of data: (12776, 22, 50)
----reconstructed_image:, Size of data: (12776, 22, 50)
----width_x:, Size of data: (12776,)
----width_y:, Size of data: (12776,)
----x:, Size of data: (12776,)
----y:, Size of data: (12776,)
--spot: spot_2:
----height:, Size of data: (12776,)
----img_max:, Size of data: (12776,)
----img_mean:, Size of data: (12776,)
----img_rec_max:, Size of data: (12776,)
----img_rec_mean:, Size of data: (12776,)
----img_rec_sum:, Size of data: (12776,)
----img_sum:, Size of data: (12776,)
----raw_image:, Size of data: (12776, 19, 40)
----reconstructed_image:, Size of data: (12776, 19, 40)
----width_x:, Size of data: (12776,)
----width_y:, Size of data: (12776,)
----x:, Size of data: (12776,)
----y:, Size of data: (12776,)
--spot: spot_3:
----height:, Size of data: (12776,)
----img_max:, Size of data: (12776,)
----img_mean:, Size of data: (12776,)
----img_rec_max:, Size of data: (12776,)
----img_rec_mean:, Size of data: (12776,)
----img_rec_sum:, Size of data: (12776,)
----img_sum:, Size of data: (12776,)
----raw_image:, Size of data: (12776, 44, 65)
----reconstructed_image:, Size of data: (12776, 44, 65)
----width_x:, Size of data: (12776,)
----width_y:, Size of data: (12776,)
----x:, Size of data: (12776,)
----y:, Size of data: (12776,)
Growth: growth_7:
--spot: spot_1:
----height:, Size of data: (12670,)
----img_max:, Size of data: (12670,)
----img_mean:, Size of data: (12670,)
----img_rec_max:, Size of data: (12670,)
----img_rec_mean:, Size of data: (12670,)
----img_rec_sum:, Size of data: (12670,)
----img_sum:, Size of data: (12670,)
----raw_image:, Size of data: (12670, 22, 50)
----reconstructed_image:, Size of data: (12670, 22, 50)
----width_x:, Size of data: (12670,)
----width_y:, Size of data: (12670,)
----x:, Size of data: (12670,)
----y:, Size of data: (12670,)
--spot: spot_2:
----height:, Size of data: (12670,)
----img_max:, Size of data: (12670,)
----img_mean:, Size of data: (12670,)
----img_rec_max:, Size of data: (12670,)
----img_rec_mean:, Size of data: (12670,)
----img_rec_sum:, Size of data: (12670,)
----img_sum:, Size of data: (12670,)
----raw_image:, Size of data: (12670, 19, 40)
----reconstructed_image:, Size of data: (12670, 19, 40)
----width_x:, Size of data: (12670,)
----width_y:, Size of data: (12670,)
----x:, Size of data: (12670,)
----y:, Size of data: (12670,)
--spot: spot_3:
----height:, Size of data: (12670,)
----img_max:, Size of data: (12670,)
----img_mean:, Size of data: (12670,)
----img_rec_max:, Size of data: (12670,)
----img_rec_mean:, Size of data: (12670,)
----img_rec_sum:, Size of data: (12670,)
----img_sum:, Size of data: (12670,)
----raw_image:, Size of data: (12670, 44, 65)
----reconstructed_image:, Size of data: (12670, 44, 65)
----width_x:, Size of data: (12670,)
----width_y:, Size of data: (12670,)
----x:, Size of data: (12670,)
----y:, Size of data: (12670,)
Growth: growth_8:
--spot: spot_1:
----height:, Size of data: (12441,)
----img_max:, Size of data: (12441,)
----img_mean:, Size of data: (12441,)
----img_rec_max:, Size of data: (12441,)
----img_rec_mean:, Size of data: (12441,)
----img_rec_sum:, Size of data: (12441,)
----img_sum:, Size of data: (12441,)
----raw_image:, Size of data: (12441, 22, 50)
----reconstructed_image:, Size of data: (12441, 22, 50)
----width_x:, Size of data: (12441,)
----width_y:, Size of data: (12441,)
----x:, Size of data: (12441,)
----y:, Size of data: (12441,)
--spot: spot_2:
----height:, Size of data: (12441,)
----img_max:, Size of data: (12441,)
----img_mean:, Size of data: (12441,)
----img_rec_max:, Size of data: (12441,)
----img_rec_mean:, Size of data: (12441,)
----img_rec_sum:, Size of data: (12441,)
----img_sum:, Size of data: (12441,)
----raw_image:, Size of data: (12441, 19, 40)
----reconstructed_image:, Size of data: (12441, 19, 40)
----width_x:, Size of data: (12441,)
----width_y:, Size of data: (12441,)
----x:, Size of data: (12441,)
----y:, Size of data: (12441,)
--spot: spot_3:
----height:, Size of data: (12441,)
----img_max:, Size of data: (12441,)
----img_mean:, Size of data: (12441,)
----img_rec_max:, Size of data: (12441,)
----img_rec_mean:, Size of data: (12441,)
----img_rec_sum:, Size of data: (12441,)
----img_sum:, Size of data: (12441,)
----raw_image:, Size of data: (12441, 44, 65)
----reconstructed_image:, Size of data: (12441, 44, 65)
----width_x:, Size of data: (12441,)
----width_y:, Size of data: (12441,)
----x:, Size of data: (12441,)
----y:, Size of data: (12441,)
Growth: growth_9:
--spot: spot_1:
----height:, Size of data: (12222,)
----img_max:, Size of data: (12222,)
----img_mean:, Size of data: (12222,)
----img_rec_max:, Size of data: (12222,)
----img_rec_mean:, Size of data: (12222,)
----img_rec_sum:, Size of data: (12222,)
----img_sum:, Size of data: (12222,)
----raw_image:, Size of data: (12222, 22, 50)
----reconstructed_image:, Size of data: (12222, 22, 50)
----width_x:, Size of data: (12222,)
----width_y:, Size of data: (12222,)
----x:, Size of data: (12222,)
----y:, Size of data: (12222,)
--spot: spot_2:
----height:, Size of data: (12222,)
----img_max:, Size of data: (12222,)
----img_mean:, Size of data: (12222,)
----img_rec_max:, Size of data: (12222,)
----img_rec_mean:, Size of data: (12222,)
----img_rec_sum:, Size of data: (12222,)
----img_sum:, Size of data: (12222,)
----raw_image:, Size of data: (12222, 19, 40)
----reconstructed_image:, Size of data: (12222, 19, 40)
----width_x:, Size of data: (12222,)
----width_y:, Size of data: (12222,)
----x:, Size of data: (12222,)
----y:, Size of data: (12222,)
--spot: spot_3:
----height:, Size of data: (12222,)
----img_max:, Size of data: (12222,)
----img_mean:, Size of data: (12222,)
----img_rec_max:, Size of data: (12222,)
----img_rec_mean:, Size of data: (12222,)
----img_rec_sum:, Size of data: (12222,)
----img_sum:, Size of data: (12222,)
----raw_image:, Size of data: (12222, 44, 65)
----reconstructed_image:, Size of data: (12222, 44, 65)
----width_x:, Size of data: (12222,)
----width_y:, Size of data: (12222,)
----x:, Size of data: (12222,)
----y:, Size of data: (12222,)
D3_para.viz_RHEED_parameter(growth='growth_2', spot='spot_2', index=100, filename=True, printing=printing_image)
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_2_spot_2_100_img,img_rec,differerce.png
../Figures/1.Fit_RHEED_spot/RHEED_untreated_162nm_growth_2_spot_2_100_img,img_rec,differerce.svg

Fig. a: RHEED spot image, b: reconstructed RHEED spot image, c: difference between original and reconstructed image for growth_2 at index 100.
img_sum=45893.00, img_max=114.00, img_mean=60.39
img_rec_sum=44505.52, img_rec_max=101.14, img_rec_mean=58.56
height=101.29, x=10.95, y=19.21, width_x=8.27, width_y_max=13.94
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9',
'growth_10', 'growth_11', 'growth_12' , 'growth_13', 'growth_14', 'growth_15', 'growth_16', 'growth_17', 'growth_18']
D3_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', metric_list=['img_sum', 'img_rec_sum', 'x', 'y', 'width_x', 'width_y'])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
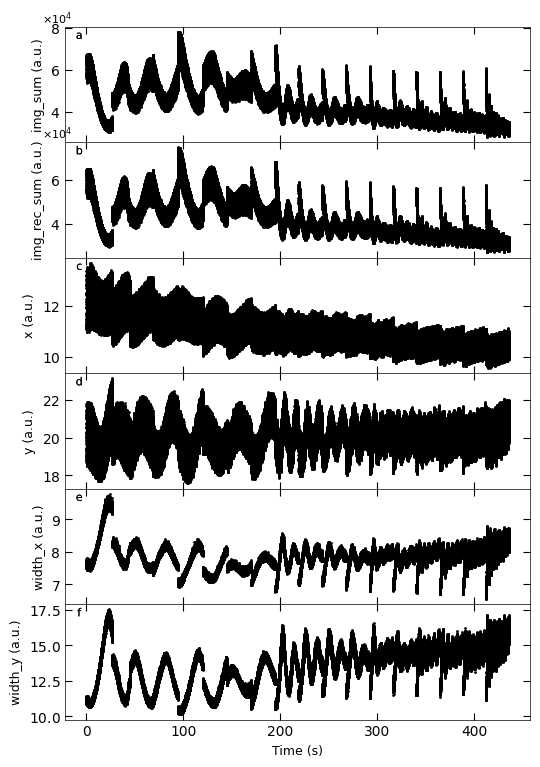
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9',
'growth_10', 'growth_11', 'growth_12' , 'growth_13', 'growth_14', 'growth_15', 'growth_16', 'growth_17', 'growth_18']
D3_para.viz_RHEED_parameter_trend(growth_list, spot='spot_2', filename='S5-untreated_162nm-RHEED_metrics', printing=printing_plot, dpi=300)
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
c:\users\yig319\lehigh university dropbox\yichen guo\predicting-pulsed-laser-deposition-srtio3-homoepitaxy-growth-dynamics-using-rheed\src\sto_rheed\Dataset.py:523: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.set_xticklabels(['' for tick in ax.get_xticks()])
../Figures/1.Fit_RHEED_spot/S5-untreated_162nm-RHEED_metrics.png
../Figures/1.Fit_RHEED_spot/S5-untreated_162nm-RHEED_metrics.svg
../Figures/1.Fit_RHEED_spot/S5-untreated_162nm-RHEED_metrics.tif
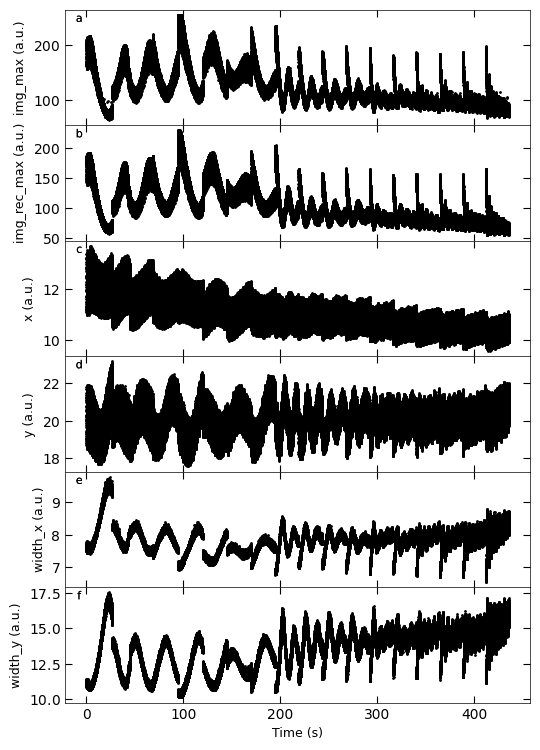
Gaussian fitted parameters in time: Fig. a: maximum intensity of original cropped RHEED spot, b: maximum intensity of resonstructed cropped RHEED spot, c: spot center in spot x coordinate, d: spot center in y coordinate, e: spot width in x coordinate, f: spot width in y coordinate.
Merged plot for RHEED intensity#
x_all_list, y_all_list = [], []
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6',
'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
x_all, y_all = D1_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
x_all_list.append(x_all)
y_all_list.append(y_all)
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9', 'growth_10', 'growth_11', 'growth_12']
x_all, y_all = D2_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
x_all_list.append(x_all)
y_all_list.append(y_all)
growth_list = ['growth_1', 'growth_2', 'growth_3', 'growth_4', 'growth_5', 'growth_6', 'growth_7', 'growth_8', 'growth_9',
'growth_10', 'growth_11', 'growth_12' , 'growth_13', 'growth_14', 'growth_15', 'growth_16', 'growth_17', 'growth_18']
x_all, y_all = D3_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
x_all_list.append(x_all)
y_all_list.append(y_all)
color_blue = (44/255,123/255,182/255)
color_orange = (217/255,95/255,2/255)
color_purple = (117/255,112/255,179/255)
three_colors = [color_blue, color_orange, color_purple]
y_max_list, y_min_list = [], []
dashed_lines_x_1 = [0, 28, 65, 84, 96, 110, 122, 134, 140, 148, 159, 166, 173,
# 182, 187, 192, 197, 207.5, 212, 216.5, 222.5,
# 227, 231.5, 235, 238.5, 243, 247, 251.5, 255.5, 259.5, 264, 268, 272, 276,
]
dashed_lines_x_2 = [23, 58, 74.5, 87, 99, 111, 118.5, 126.5, 135.5, 143, 151]
dashed_lines_x_3 = [1, 39, 66, 98, 130, 164, 198, 210, 222, 235, 248, 260, 272, 284, 297]
dashed_lines_x_list = [dashed_lines_x_1, dashed_lines_x_2, dashed_lines_x_3]
xlim_list = [(-8, 308), (-7, 262), (-12, 450)]
fig, axes = layout_fig(graph=3, mod=1, figsize=(8, 7), layout='tight')
for ax, x_all, y_all, color, dashed_lines_x, xlim in zip(axes, x_all_list, y_all_list, three_colors, dashed_lines_x_list, xlim_list):
# normalize the intensity
y_min_list.append(np.min(y_all))
y_max_list.append(np.max(y_all))
y_all = NormalizeData(y_all, lb=np.min(y_all), ub=np.max(y_all))
ax.plot(x_all, y_all, color='black', linewidth=1)
for x in dashed_lines_x:
ax.plot([x, x], [np.min(y_all-0.05), np.max(y_all+0.05)], linestyle='--', linewidth=1, color=color)
ax.set_xlim(xlim)
ax.set_ylim(-0.1, 1.1)
Viz.set_labels(ax, xlabel='Time (s)', ylabel='Intensity (a.u.)', yaxis_style='float')
plt.savefig('../Figures/1.Fit_RHEED_spot/summarized_spot_2_sum.png', dpi=600, bbox_inches='tight', transparent=True)
plt.show()

x_all_list, y_all_list = [], []
growth_list = ['growth_1', 'growth_2', 'growth_3']
x_all, y_all = D1_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
y_all = y_all[(x_all>28) & (x_all<65)]
x_all = x_all[(x_all>28) & (x_all<65)]
x_all_list.append(x_all)
y_all_list.append(y_all)
growth_list = ['growth_1', 'growth_2', 'growth_3']
x_all, y_all = D2_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
y_all = y_all[(x_all>23) & (x_all<60)]
x_all = x_all[(x_all>23) & (x_all<60)]
x_all_list.append(x_all)
y_all_list.append(y_all)
growth_list = ['growth_1', 'growth_2']
x_all, y_all = D3_para.load_multiple_curves(growth_list=growth_list, spot='spot_2', metric='img_rec_sum')
y_all = y_all[(x_all>1) & (x_all<39)]
x_all = x_all[(x_all>1) & (x_all<39)]
x_all_list.append(x_all)
y_all_list.append(y_all)
fig, axes = layout_fig(graph=3, mod=1, figsize=(3, 7), layout='tight')
for ax, x_all, y_all, y_min, y_max, color, dashed_lines_x in zip(axes, x_all_list, y_all_list, y_min_list, y_max_list, three_colors, dashed_lines_x_list):
x_all = x_all - x_all[0]
y_all = NormalizeData(y_all, lb=y_min, ub=y_max)
ax.plot(x_all, y_all, color='black', linewidth=1)
ax.set_xlim(-2, 40)
ax.set_ylim(-0.1, 1.1)
Viz.set_labels(ax, xlabel='Time (s)', ylabel='Intensity (a.u.)', yaxis_style='float')
plt.savefig('../Figures/1.Fit_RHEED_spot/summarized_single_unit_cell_growth.png', dpi=600, bbox_inches='tight', transparent=True)
plt.show()
